In this issue, I’m going to walk through a primer about autoloading within WordPress plugins.
Especially since it is a concept that will hopefully be included with WordPress soon.
When you implement an autoloading solution, you no longer need to use any hardcoded require or include statements.
There’s no need for a full file that lists out includes for every file in your plugin so they are loaded when the plugin is active.
Unfortunately, with a lot of plugins that I’ve seen, developers are still manually loading individual files.
Too many WordPress plugin developers don’t know how to use autoloading
Most developers haven’t added autoloading to their plugins because:
- They don’t understand the benefit
- They think it will slow down active development
- They think adding an autoloader would require too much configuration
After this issue, you will understand why an autoloader is important and how it can actually speed up development.
Let’s dig in:
What is autoloading in PHP?
Every active plugin in a WordPress site is loaded when a user sends a request.
When the request comes in, plugins need to load code into memory that may need to be referenced in the future.
Most of the time, a large portion of your codebase isn’t even used.
Do you need to load everything for every WP_CLI command? Probably not
Whenever the cron runs? Nope
During the ajax heartbeat or when WordPress autosaves? Most likely not
If you’re doing a bunch of requires, require_onces, includes, or include_onces you’re loading a lot of code that may not be necessary.
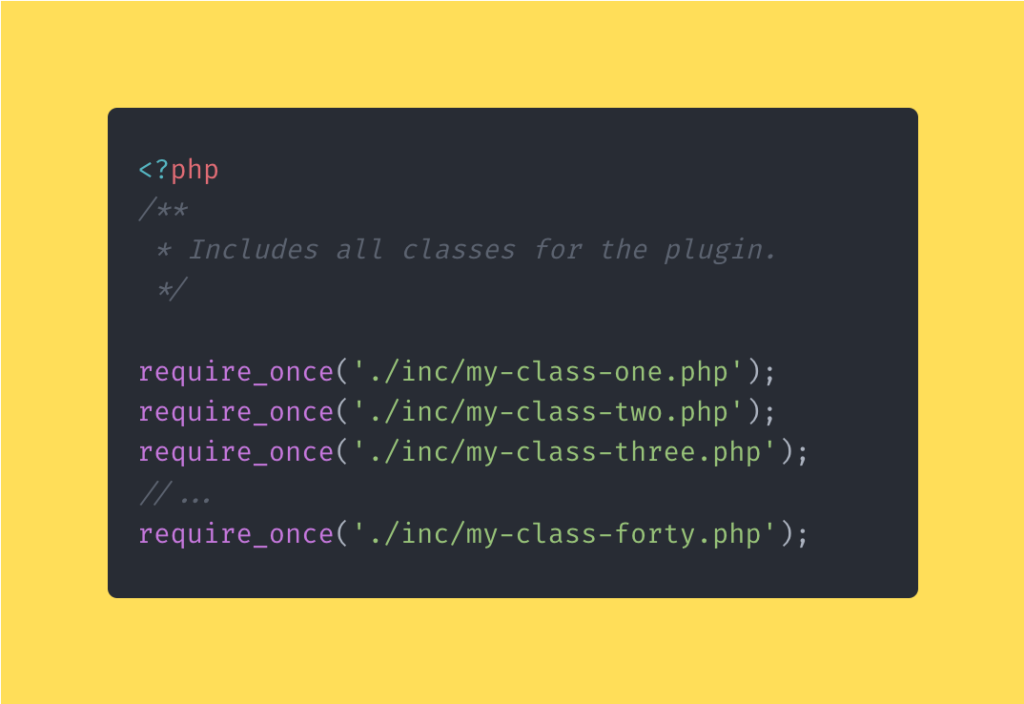
Instead, autoloading provides a mechanism that allows files to be loaded on-the-fly and only when they’re actually needed.
This makes your code smarter and only runs what’s actually necessary when a request comes in.
Using composer, and a few lines of code, you can turn that example above into something like this:
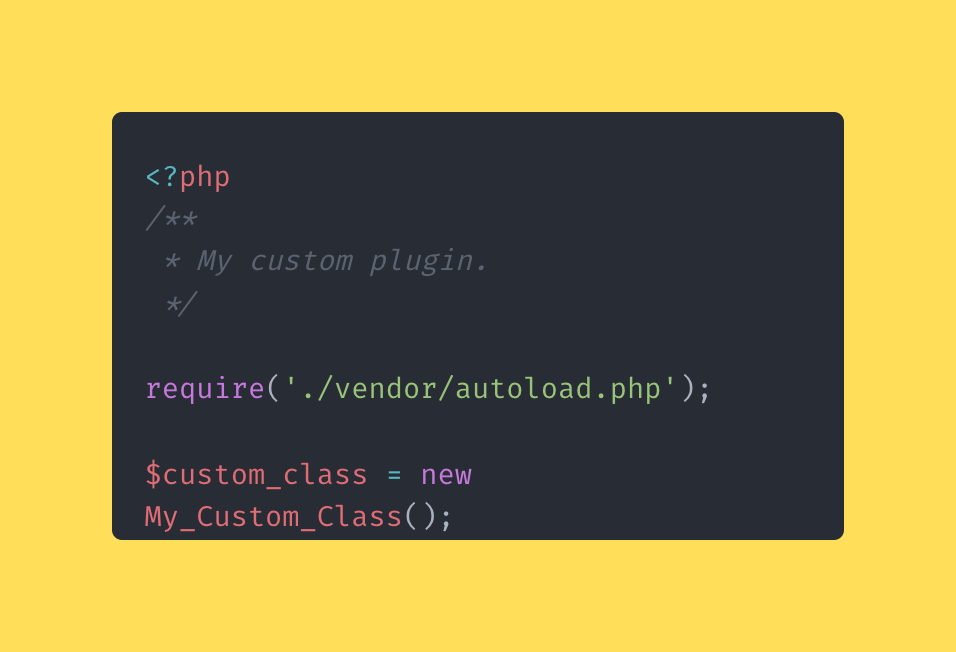
A quick note on namespaces
At the core, the autoloading system relies on Namespacing.
If you’re not familiar, namespacing is a way to encapsulate your code, or a way to group it together in a logical structure.
You can define a specific scope for all the code in your plugin.
This helps you avoid the issue of trying to load a class name that is already in the global namespace.
If you want to read more into what Namespaces do and how they work, take a look through the PHP documentation.
So how does autoloading work?
When you instantiate a class in PHP, much like a function, the class must be defined before you can use it.
An autoloader registers a root namespace that maps to a certain directory in your plugin.
In other words, TAR\Plugin
points the autoloader to the src/
directory.
Now the autoloader knows where to find the class it needs based on your file structure.
Let’s say you have a Numbers
class in your plugin within the src
directory:
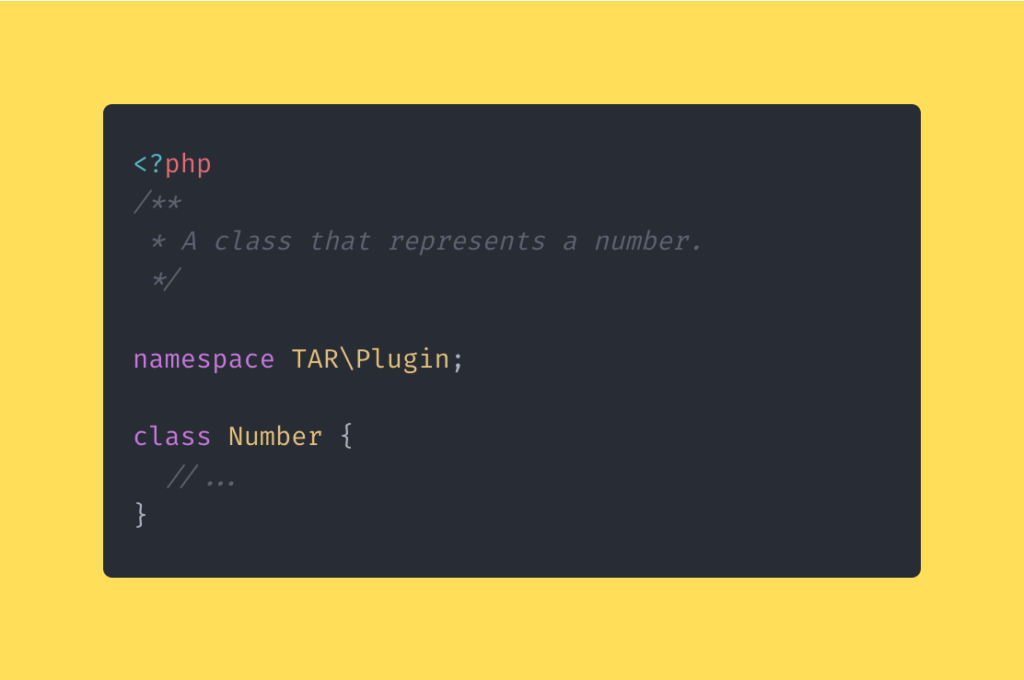
When you try to use the class, the autoloader will traverse the namespace of the class you’re referencing and match it to the folder structure.
When you instantiate TAR\Plugin\Numbers
the autoloader will look in src/Numbers.php
for the definition of the class. If that file exists, it will automatically be loaded and ready for you to reference it.
Now, instead of loading all of your class files with require
or include
you can include an autoloader and you don’t have to worry about including a class before you call it.
You just set up an autoloader (with a library like Composer), and as long as you have the correct file structure and namespace, you’re class files will be loaded automatically.
If you’d like to actually implement an autoloader in your plugin, check out an earlier issue of this newsletter that helps you get started with Composer.
In Summary
Autoloaders help reduce your workload because they automatically load your class definitions for you. Provided you have the correct folder & namespace set up.
Once you set up an autoloader, you don’t typically have to think about it again and you don’t have to manage loading a class before you try to use it.
I set up an autoloader with every plugin and I rarely think about what’s going on behind the scenes anymore.