This week, I’m going to show you one of the best ways I’ve found to write quality hook names for your plugin’s actions & filters.
I’ve been working on a few plugins recently where I needed to implement some hooks so users could override values in an API request.
First, a little context: I didn’t start the plugin, but I was brought on to help complete it.
After looking through the codebase, I found that all the hooks were using the WordPress suggested naming conventions.
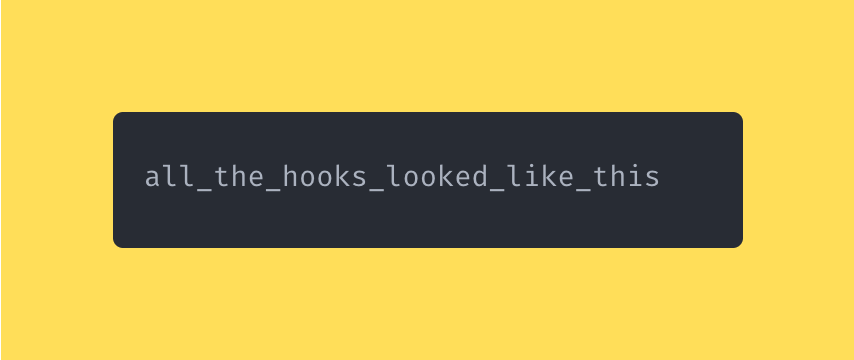
At first, it wasn’t an issue, but as we added features to the plugin I realized that naming hooks like this quickly got confusing:
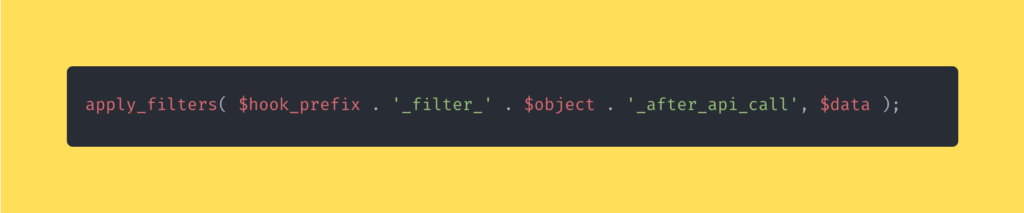
That’s when I remembered I saw a different convention in the Advanced Custom Fields plugin that really resonated with me:
Naming conventions are not laws, but guidelines
There’s a lot of merit to why the hooks in WordPress are all snake-case (a string separated with underscores: snake_case).
Instead, use slashes and namespace your hook names:
- Improve readability of hooks within code
- Namespacing is common in PHP codebases
- Increase the specificity of similar hooks and avoid name collisions
Naming hooks this way is a great step in the right direction.
Namespacing Is A Consistent PHP Convention
In the process of writing your plugin (or thinking about writing one), you’ve probably seen some namespacing in WordPress core.
Namespacing is a widely accepted convention because it improves the logical grouping of code in a codebase.
This helps developers identify collections of features and similar functionality.
When determining your plugin’s hook names, a namespaced approach is best for two reasons:
- It helps you identify what the hook does just by its namespace
- It helps provide protection against collisions because no one else should be using your namespace
Lets take a look at an example:
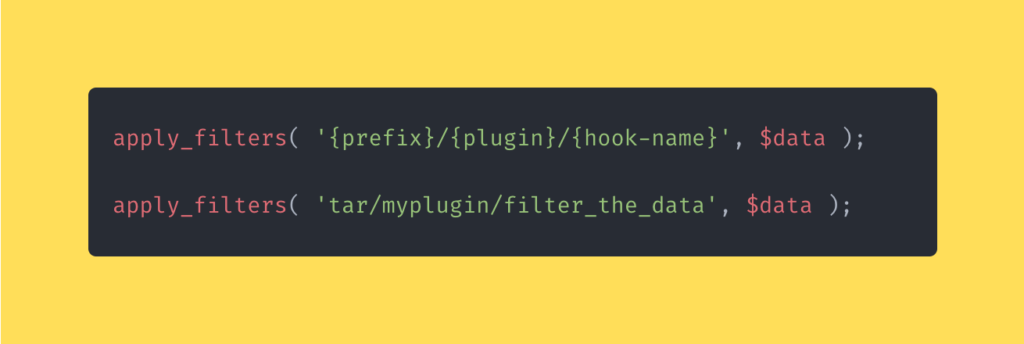
Already, just by looking at that filter name, you already know that it’s related to my plugin (tar is my initials so I often use it as a prefix) and it filters some kind of data.
Just like namespacing, the domain gets smaller as you move from left to right:
- tar – All of my plugins
- myplugin – One specific plugin
- filter_the_data – The actual hook function
Provide Specificity In Your Hook Names
Additionally, you can use this naming structure to add specificity to show what the hook does in your codebase.
From our example above, we can add another level:
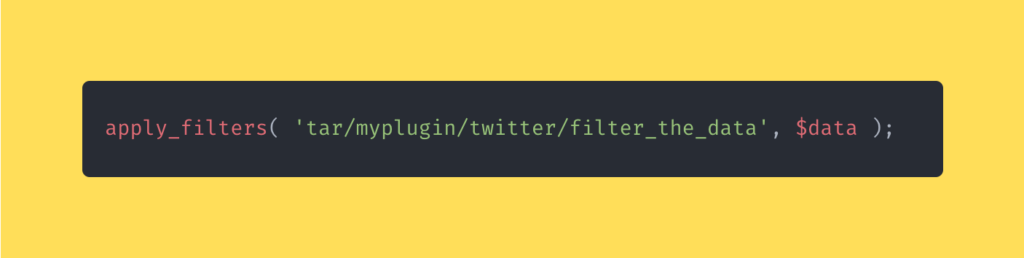
Now, with that extra level, you know that it’s filtering some kind of data for Twitter.
It just adds a little more context and specificity to what the hook does.
It also further guards you against having the same hook name as another plugin or even your own hook names.
For example, what if we also had to filter data for instagram? We could just add that to the hook name:
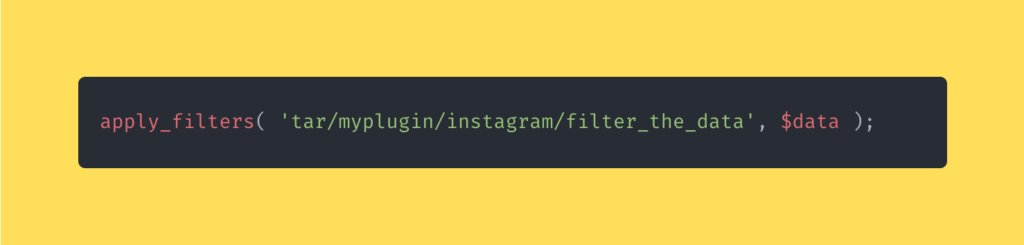
Since the hook name is just a string, you also have no limit to the amount of levels you can add (although, you should probably keep it to 1 or 2).
Improve the Readability Hooks Within Your Codebase
Readable hooks are always a good thing, especially if you have a large codebase with many available hooks.
Using /
, you make your hook names easier to consume than something like this:
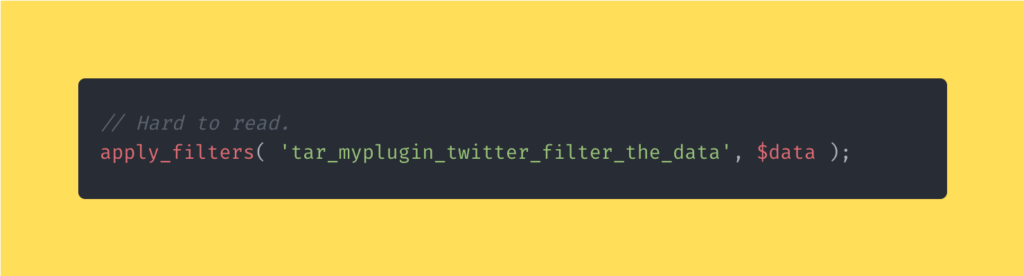
Without those slashes, hook names are harder to read.
What’s the prefix?
tar?
tar_myplugin?
As an added benefit from the first example I showed, interpolating variables in your hook name is also easier to parse:
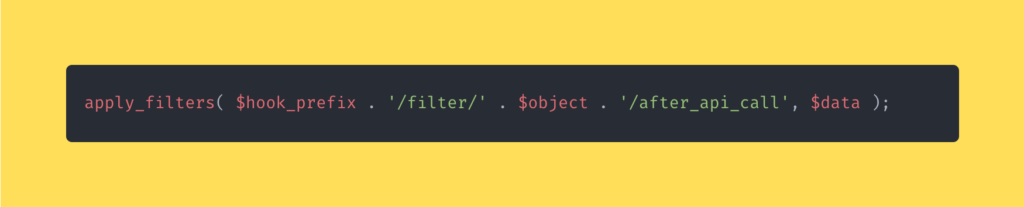
In Summary
Hook names in WordPress are just strings which can be anything, but have a convention to be snake_cased.
Using a namespace approach, your hook names are more readable, specific, and consistent.
When you provide hooks in your plugin, how do you format them?
Do you already use this type of naming structure?