As a plugin developer, you are adding functionality that is processed each time a user sends a request to the server.
You are probably aware of several hooks outlined in the WordPress request lifecycle, but there is one specific hook that doesn’t get as much attention as I think it should.
Whenever you need to handle a slow task, do it in the background
Use the shutdown hook as a simple way to do background processing:
- Perform a complex calculation and save it to a post’s meta
- Generate new transients to prime a cached resource
- Process items in a queue stored in the database
None of these actions should cause the user to wait during the request, but help with the overall site experience.
Here’s how you can start using it today:
The WordPress Request Lifecycle
First, let’s start with a brief overview of the WordPress request lifecycle.
For every request a user makes to a WordPress site, the request triggers WordPress to load through accessing the wp-load.php
file.
Once WordPress is loaded, it runs through all registered hooks and builds the correct page the user requested, handles any necessary actions required by that page and sends the resulting html & css to the browser.
After everything is done, just before all PHP execution is stopped, WordPress runs one final hook: shutdown
If you want to run code after the user’s browser receives the finished request but just before execution is stopped, you can use the shutdown hook. It’s great when you want to continue processing some PHP code without slowing down the user’s experience.
Register a function with the ‘Shutdown’ hook
Set up a new action and register it with your function:
function swpd_prime_image_cache() {
// prime your image cache for the next page load.
}
add_action( 'shutdown', 'swpd_prime_image_cache' );
Now, whenever WordPress handles a request and reaches the shutdown step, your function—priming an image cache—will be executed.
The shutdown hook is run after WordPress returns the request to the user, so at that point, it’s essentially running in the background after the page is rendered.
Warning: Caveats
If a user has an error with their site, it’s possible that a fatal error kills the request before the ‘shutdown’ action is actually called.
You should only rely on shutdown for things that aren’t critical or can be executed again in the next request.
Notable Plugins Utilizing The Shutdown Hook
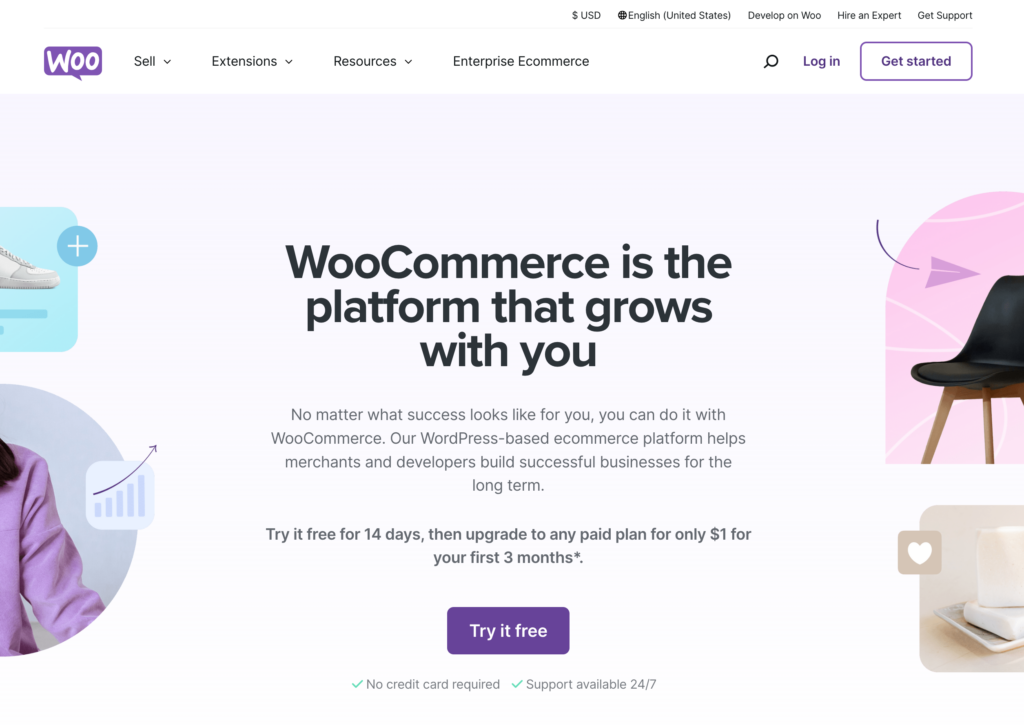
WooCommerce
The action scheduler library that powers queueing background actions and handles millions of ecommerce notifications and purchases does all this by hooking into the shutdown action.
Normally, the library looks for events to process during a once-per-minute cron, but it also checks during the shutdown
hook (in the admin) to catch any events that were created during the request.
In this case, events are stored in the database for later processing and will either be handled via cron or after the request has finished. Both times the user is unaware.
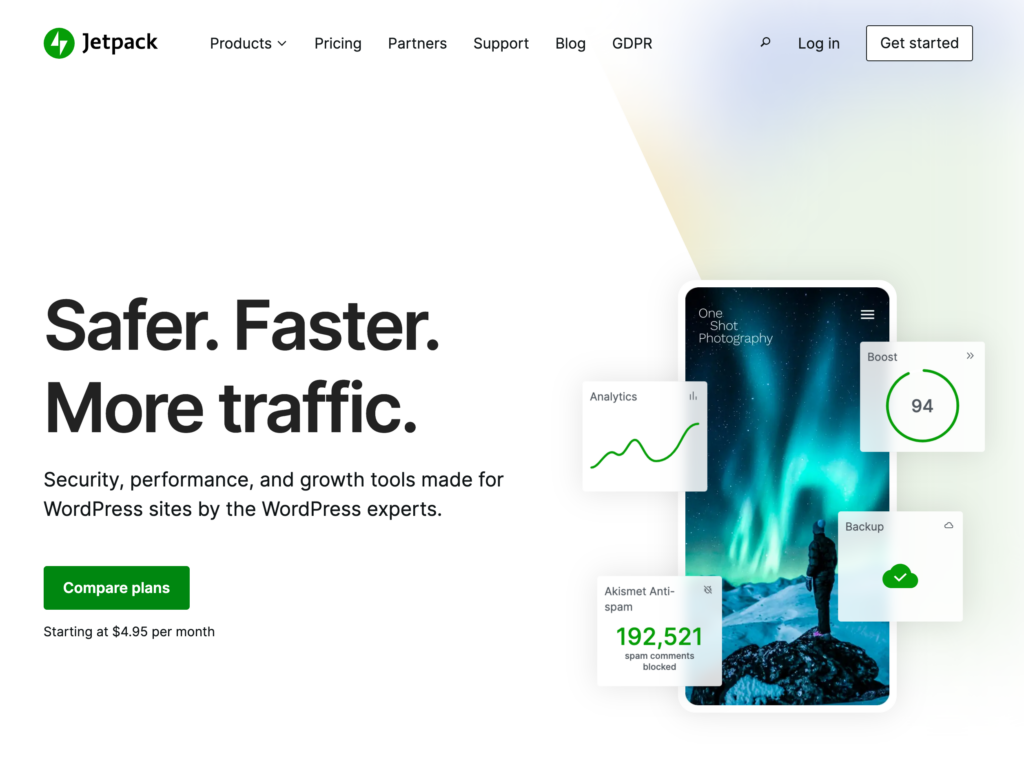
Jetpack
There are quite a few places where Jetpack relies on the shutdown
hook. Most of these instances are handling uncaught errors, purging caches, or calculating stats.
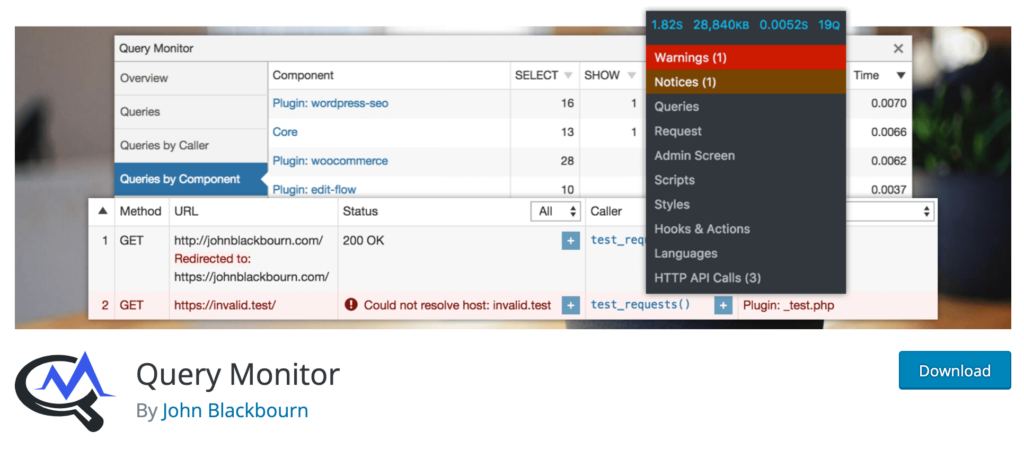
Query Monitor
The shutdown
hook is used in several places, but most notably to handle calculating the request processing time.